How to develop a simple calculator using C Sharp (C#) Visual Studio 2005



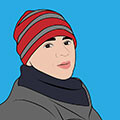
This is my second calculator I developed using C sharp Visual Studio 2005 as the first one was a basic one. Well it is easy to code programs(basics) using Visual Studio as I always thought it would be quite tough. Well a bit introduction on Visual Studio 2005. Visual Studio is a suite of products developed by Microsoft that allow one to build .NET applications, which execute within the .NET Framework. Visual Studio 2005 supports four .NET languages:
- Visual Basic .NET [Designed for rapid application development]
- Visual C#.NET [A language that combines Java and C++]
- Visual J# .NET [Microsoft's versions of Java which runs in.NET Framework]
- Visual C++ .NET[Microsoft's Version of C++ that can be used for applications that need high performance.]
- Microsoft Development Environment [The Integrated Development Environment[IDE] that you use for developing applications
- Microsoft SQL Server 2005 Express or Developer Edition[includes the unique Application XCopy feature, and networking and security that differ from other SQL Server 2005 editions]
Well let's start now with developing a simple calulator using C# as your programming language.
Step 1: Launch your Microsoft Visual Studio 2005.
Step 2: Click on File, New Project.
Step 3 : Under "Project types" select Visual C#, select "Windows Applications" and rename it from "WindowsApplication1" to "Calculator"
Step 4 : Click OK.
Once you're there now locate your "toolbox" as you'll need it to drag and drop your controls onto your pages / forms. If you can't find it on the left hand side then you can simply press "control+alt+x" or click on view then toolbox.
You'll need 18 buttons(you can have more). 0-9 will comprise of number buttons, you'll have one "+" sign, one "-" sign, one "*" sign, one "/" sign, one "+/-" sign, one "." sign one "clear" button, one "answer" button, and lastly you'll need to have one text box to display results of your calculations.
You'll notice "Form1" on top left of the form. If you want to rename it to "Calculator" just click on the form and on the right hand side you'll notice properties. Search for text and rename it. After doing that now, rename all the buttons to their respective names.
Now coming to the coding process its very easy. It needs logic and simple English words.
Double click on the button "1" and write the following command:
textBox1.Text = textBox1.Text + "1";
Now code all your buttons(numbers only) you just need to increment it by one.
For e.g the 2 button should be code like this
textBox1.Text = textBox1.Text + "2";
The code for the calculator is as follows ;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Linq;
namespace Calculator
{
public partial class Calculator : Form
{
bool plus = false;
bool minus = false;
bool multiply = false;
bool divide = false;
bool equal = false;
public Calculator()
{
InitializeComponent();
}
private void CheckIfequal()
{
if (equal)
{
textBox1.Text = "";
equal = false;
}
}
private void button1_Click(object sender, EventArgs e)
{
CheckIfequal();
if (equal)
equal = false;
textBox1.Text = textBox1.Text + "1";
}
private void button2_Click(object sender, EventArgs e)
{
CheckIfequal();
textBox1.Text = textBox1.Text + "2";
}
private void button3_Click(object sender, EventArgs e)
{
CheckIfequal();
textBox1.Text = textBox1.Text + "3";
}
private void button4_Click(object sender, EventArgs e)
{
CheckIfequal();
textBox1.Text = textBox1.Text + "4";
}
private void button5_Click(object sender, EventArgs e)
{
CheckIfequal();
textBox1.Text = textBox1.Text + "5";
}
private void button6_Click(object sender, EventArgs e)
{
CheckIfequal();
textBox1.Text = textBox1.Text + "6";
}
private void button7_Click(object sender, EventArgs e)
{
CheckIfequal();
textBox1.Text = textBox1.Text + "7";
}
private void button8_Click(object sender, EventArgs e)
{
CheckIfequal();
textBox1.Text = textBox1.Text + "8";
}
private void button9_Click(object sender, EventArgs e)
{
CheckIfequal();
textBox1.Text = textBox1.Text + "9";
}
private void button10_Click(object sender, EventArgs e)
{
CheckIfequal();
textBox1.Text = textBox1.Text + "0";
}
private void buttonDecimal_Click(object sender, EventArgs e)
{
CheckIfequal();
if (textBox1.Text.Contains("."))
{
return;
}
else
{
textBox1.Text = textBox1.Text + ".";
}
}
private void buttonPlusMinus_Click(object sender, EventArgs e)
{
CheckIfequal();
if (textBox1.Text.Contains("-"))
{
textBox1.Text = textBox1.Text.Remove(0, 1);
}
else
textBox1.Text = "-" + textBox1.Text;
}
private void buttonPlus_Click(object sender, EventArgs e)
{
CheckIfequal();
if (textBox1.Text == "")
{
return;
}
else
plus = true;
textBox1.Tag = textBox1.Text;
textBox1.Text = "";
}
private void buttonAnswer_Click(object sender, EventArgs e)
{
equal = true;
if (plus)
{
decimal dec = Convert.ToDecimal(textBox1.Tag)
+ Convert.ToDecimal(textBox1.Text);
textBox1.Text = dec.ToString();
}
if (minus)
{
decimal dec = Convert.ToDecimal(textBox1.Tag)
- Convert.ToDecimal(textBox1.Text);
textBox1.Text = dec.ToString();
}
if (multiply)
{
decimal dec = Convert.ToDecimal(textBox1.Tag)
* Convert.ToDecimal(textBox1.Text);
textBox1.Text = dec.ToString();
}
if (divide)
{
decimal dec = Convert.ToDecimal(textBox1.Tag)
/ Convert.ToDecimal(textBox1.Text);
textBox1.Text = dec.ToString();
}
}
private void buttonMinus_Click(object sender, EventArgs e)
{
CheckIfequal();
if (textBox1.Text == "")
{
return;
}
else
minus = true;
textBox1.Tag = textBox1.Text;
textBox1.Text = "";
}
private void buttonMultiply_Click(object sender, EventArgs e)
{
CheckIfequal();
if (textBox1.Text == "")
{
return;
}
else
multiply = true;
textBox1.Tag = textBox1.Text;
textBox1.Text = "";
}
private void buttonDivide_Click(object sender, EventArgs e)
{
CheckIfequal();
if (textBox1.Text == "")
{
return;
}
else
divide = true;
textBox1.Tag = textBox1.Text;
textBox1.Text = "";
}
private void buttonClear_Click(object sender, EventArgs e)
{
plus = minus = multiply = divide = equal = false;
textBox1.Text = "";
textBox1.Tag = "";
}
}
}
It should be working however if you're having any problems just drop me a comment and i'll be glad to help! Enjoy your new calculator :D
9 Replies
Your original code did not compile, it was missing the CheckIfequal() method (maybe something got lost in Wordpress or during the transfer). Nevermind, I have added this function and updated your code above to make it work. I haven't tested the functionality of the program though but I assume it works :)
Okay thank you.. Maybe it got lost, because it compiled successfully at my place and everything works. :)
Hi, I want to write in Visual Studio as a code the following:
t3 * sin ^4 (fi) where t3 and fi are variables type double.
Would you mind to tell me?Thank you!
Math.Sin(a) will give you the sine of an angle and it needs to be in radians, if you have the variable a in degrees, then multiply it by Math.PI/180 to get the value in radians.
Math.Pow(x,y) is straightforward 2^3 will be Math.Pow(2,3) which will give you 2x2x2 = 8.
Thanks for sharing.
Its kinda rare to see a Mauritian share his knowledge about programming.
By the way for those interested in developping android apps, please check out Titanium or phonegap , those are very interesting indeed. they allow you to program android apps with nothing but javascript, html and css. i will not go in depth on this topic , it can take ages :P lol..
and yea best editor for developping android apps is eclipse , however titanium editor is not bad too.
Do you do android apps? I'm starting to make some. I ditched away Visual Studio long time ago.
No, not android for the time being, later don't know. Btw why you "ditched away Visual Studio" ??
Use the 2012 ultimate edition, lots of interesting features in that..
In the visual studio professional 2010 & 2012 u can create Android apps with a name called Droid application development
Similar topics for you

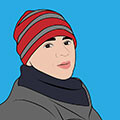


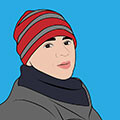

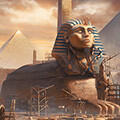

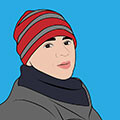
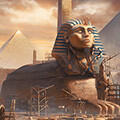
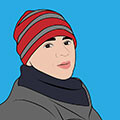



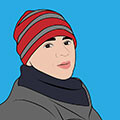
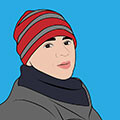


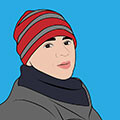